Wildcard Types
Wildcard types are type parameters defined using the wildcard symbol ?. The wildcard ? by itself represents all types. The parameterized type List<?> represents a list of all types, whereas the concrete parameterized type List<Integer> only represents a list of Integer. In other words, a wildcard type can represent many types. Therefore, a parameterized type that has wildcard types as actual type parameters can represent a family of types, in contrast to a concrete parameterized type that only represents itself. The wildcard types provided in Java represent four subtype relationships that are summarized in Table 11.1.
Wildcard types provide the solution for increased expressive power to overcome the limitations discussed earlier when using generics in Java, but introduce limitations of their own as to what operations can be carried out on an object using references of wildcard types. We will use the class Node<E> in Example 11.2, p. 568, as a running example to discuss the use of wildcard types.
Table 11.1 Summary of Subtyping Relationships for Generic Types
Name | Syntax | Semantics | Description |
Subtype covariance | ? extends Type | Any subtype of Type (including Type) | Bounded wildcard with upper bound |
Subtype contravariance | ? super Type | Any supertype of Type (including Type) | Bounded wildcard with lower bound |
Subtype bivariance | ? | All types | Unbounded wildcard |
Subtype invariance | Type | Only type Type | Type parameter/argument |
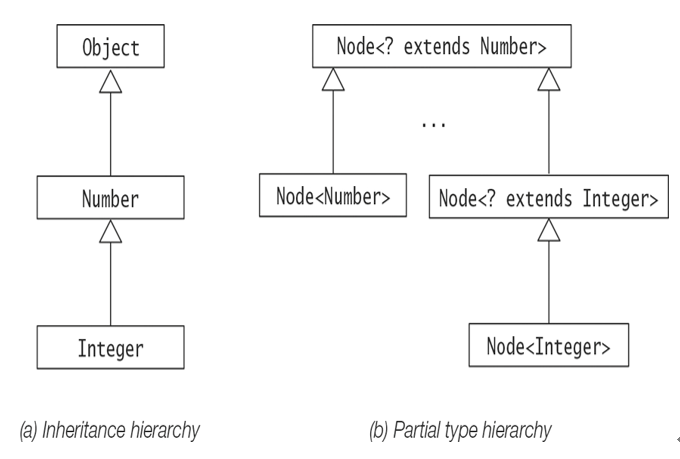
Figure 11.3 Partial Type Hierarchy for Node<? extends Number>
The wildcard type ? extends Number denotes all subtypes of Number, and the parameterized type Node<? extends Number> denotes the family of invocations of Node<E> for types that are subtypes of Number. Figure 11.3 shows a partial type hierarchy for the parameterized type Node<? extends Number>. Note that the parameterized type Node<? extends Integer> is a subtype of the parameterized type Node<? extends Number>, since the wildcard type ? extends Integer represents all subtypes of Integer, and these are also subtypes of Number.
Node<? extends Integer> intSubNode = new Node<Integer>(100, null);
Node<? extends Number> numSupNode = intSubNode;
Leave a Reply